I need help making cards like these:
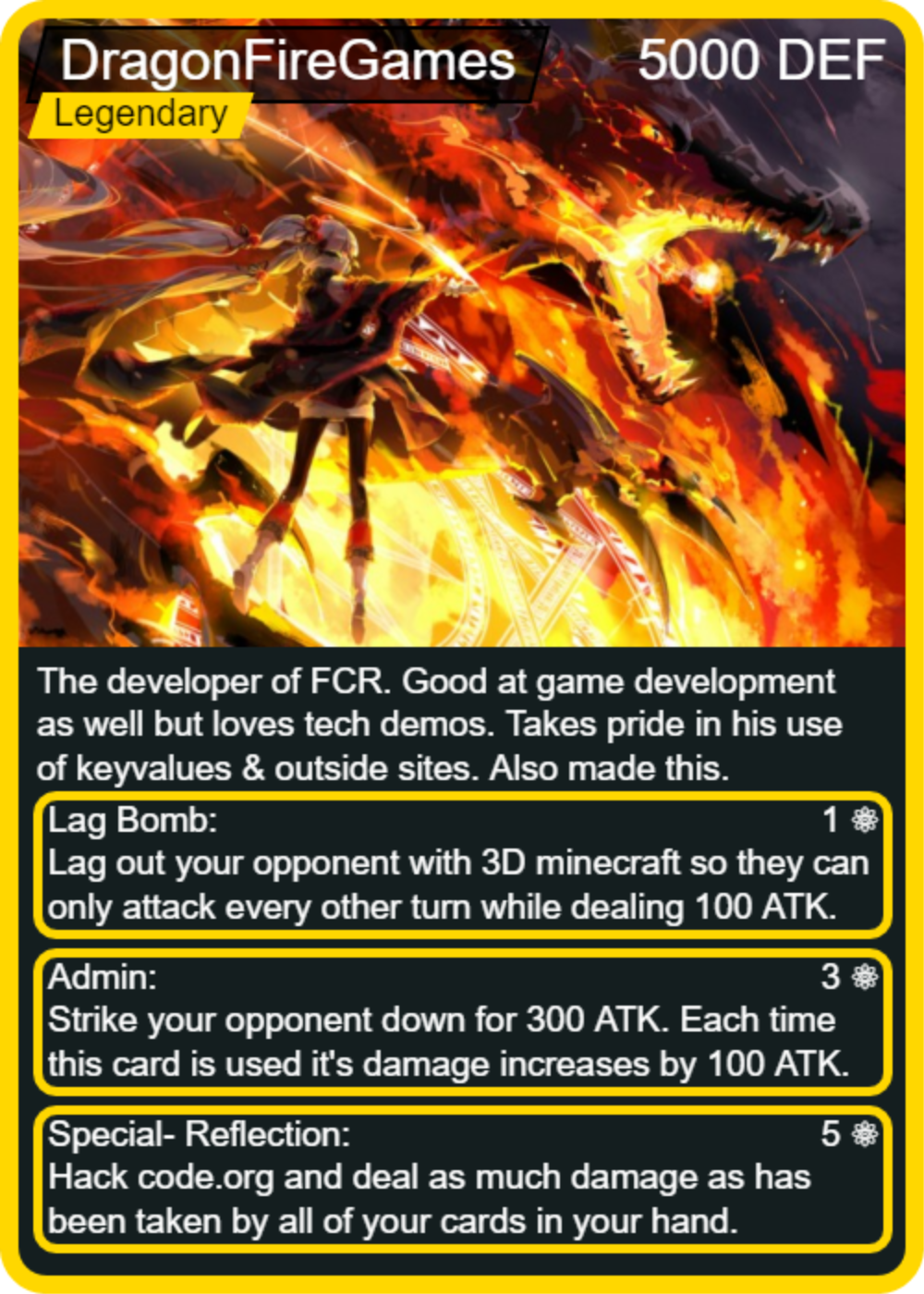
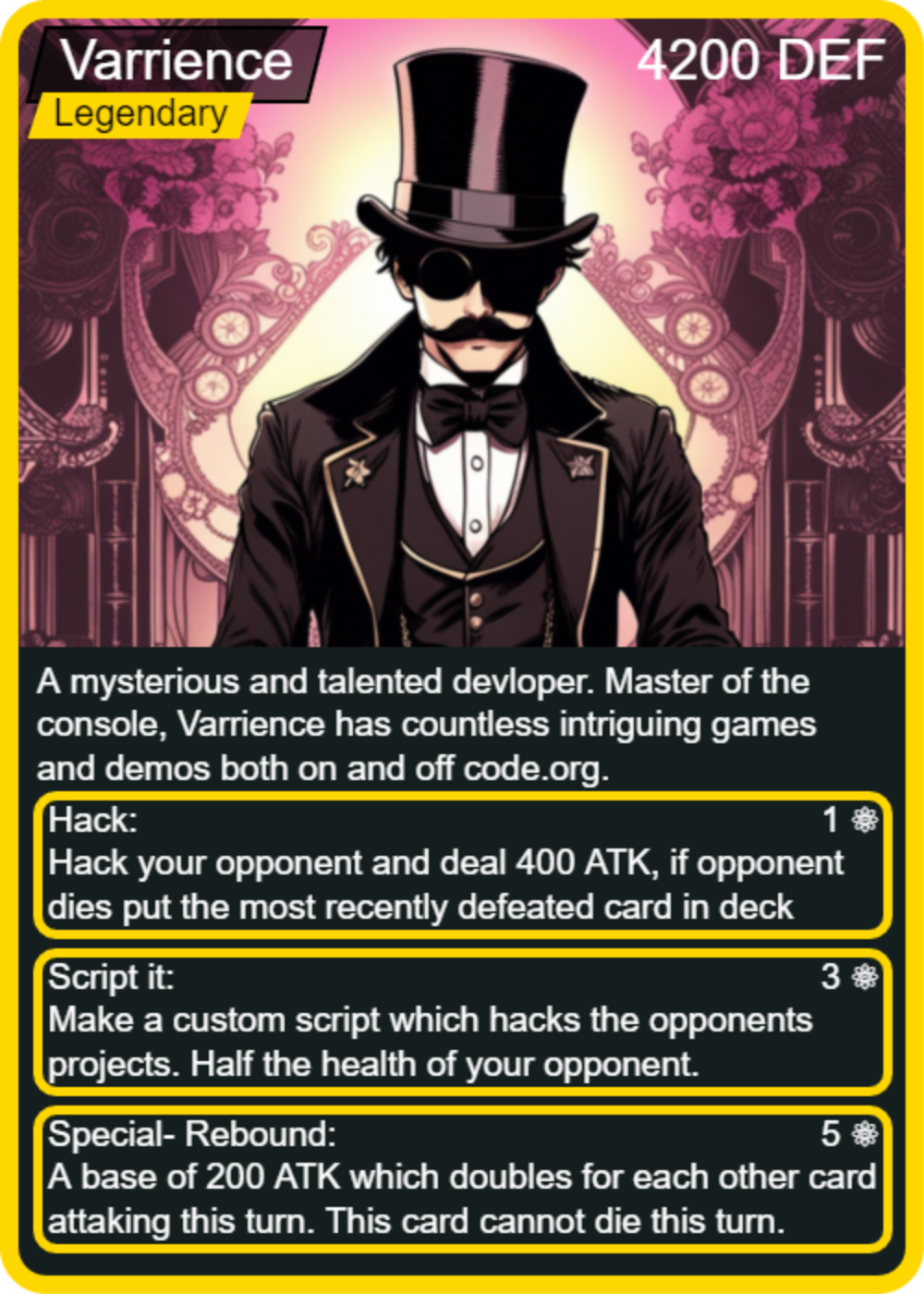

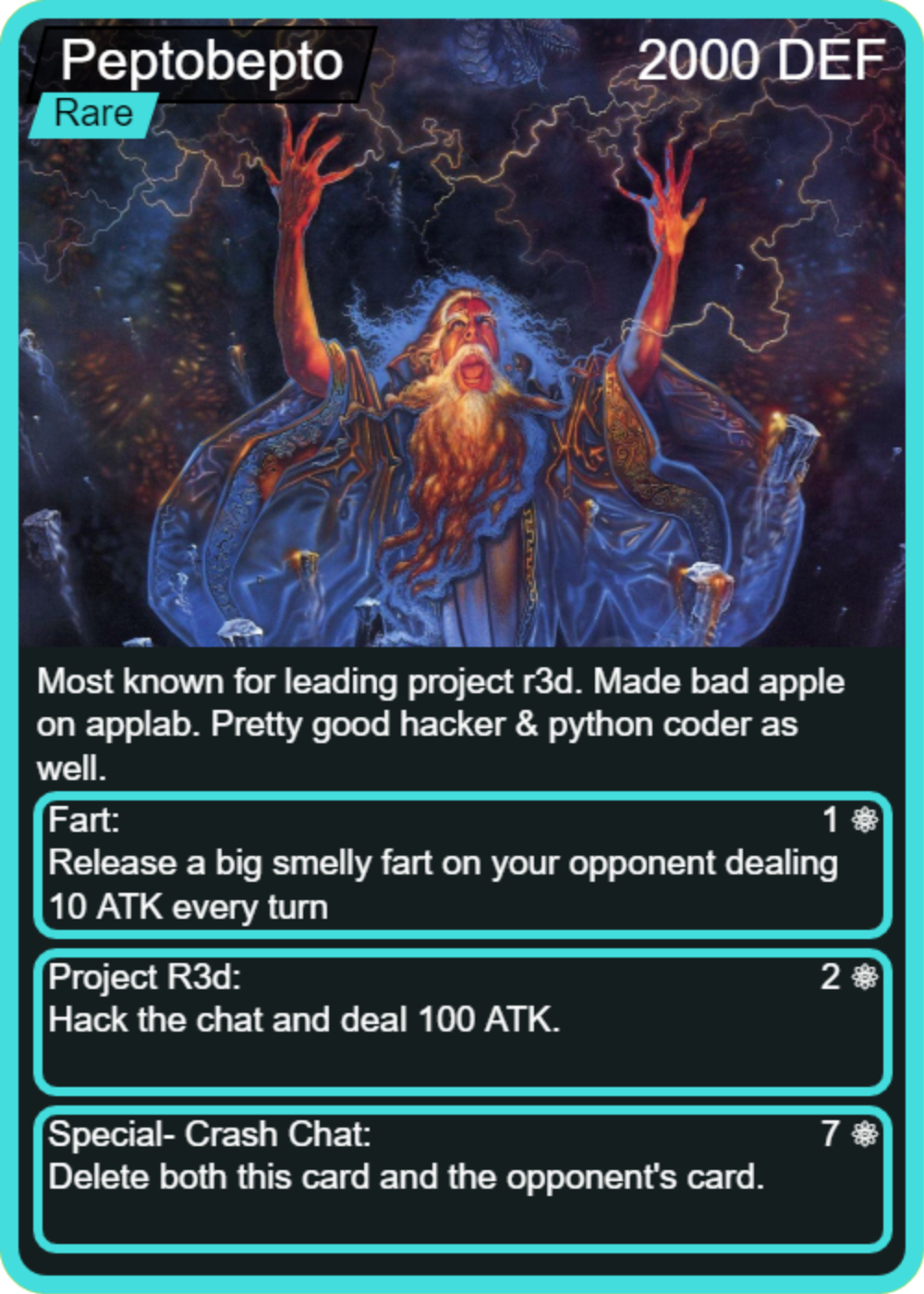
Create your card using this project by remixing one of our cards:
https://studio.code.org/projects/gamelab/prA3iibnYXDFHL5Jl9SlA2Y7djtm-Huqy78EiV4f7d4/view
Send me the result in code like this:
createCard({
name:"DragonFireGames",
rarity:"Legendary",
health:5000,
image:"https://mcdn.wallpapersafari.com/medium/75/47/2gJuCk.jpg",
offx: 1,
offy: 0,
description: "The developer of FCR. Good at game development as well but loves tech demos. Takes pride in his use of keyvalues & outside sites. Also made this.",
hpcol: 255,
moves:[{
title:"Lag Bomb",
description:"Lag out your opponent with 3D minecraft so they can only attack every other turn while dealing 100 ATK.",
cost:1,
cooldown:1,
use:function(opp) {
opp.health -= 100;
opp.status.push("Laggy");
}
},{
title:"Admin",
description:"Strike your opponent down for 300 ATK. Each time this card is used it's damage increases by 100 ATK.",
cost:3,
cooldown:1,
use:function(opp) {
opp.admindmg = opp.admindmg || 500;
opp.health -= opp.admindmg;
opp.admindmg += 100;
}
},{
title:"Special- Reflection",
description:"Hack code.org and deal as much damage as has been taken by all of your cards in your hand.",
cost:5,
cooldown:2,
use:function(opp,self) {
opp.health += self.health-self.parent.health;
}
}],
});
Don't worry about the use functions, but if you can make them please do. I will personally balance your submissions according to your rank on the forum as well, so don't just create op cards, create unique, personalized cards that reflect yourself & who's abilities reflect your skills, projects, and other unique talents.
The card game works with a 20 card deck where each player has a hand of 4 cards. When a card is defeated, they draw another card randomly from their deck into their hand. Each turn a player can make as many moves as they want, and end the turn when they are done or when they run out of ⚛ points.
Every 10 turns the number of ⚛ increases by 1. The starting amount is 1⚛ per turn.
Your rarity will also be the same as in this: https://gamelab.freeflarum.com/d/1438-ranking-system