This may not cover every piece of Java you can use in code.org but this the main stuff I've seen in others' programs.
- Short Hand if else statements. These are used to shorten if else statements in your code.
A shorthand if else looks like this: variable = (operator/condition) ? ifTrue : ifFalse;
For example, if I wanted to write this:
if (skibidi == 20){
console.log("Get Fanum Taxed");
} else {
console.log("The Fanum Taxer Thinks Ur Not Skibidi");
}
I could simplify that and write:
var skibidi = 20;
var output = (skibidi == 20) ? "Get Fanum Taxed" : "The Fanum Taxer Thinks Ur Not Skibidi";
console.log(output);
- The Java Switch. This is basically another way to write multiple
else
statements within the same "block" of code. The Java switch evaluates multiple cases that are all associated with the same expression. This is how you write a Java switch statement:
switch(skibidi){
case 1:
//Code stuff
break;
case 2:
//Code stuff
break;
default:
//Code stuff
break;
}
Both default
and break;
are optional but here is what they do:
default
is the code executed if each case
does not match.
break;
is to stop the evaluation of each case
. This is usually used after a case
matches the expression given.
- Java
continue;
. continue;
is used in loops to break;
a certain outcome while still continuing the loop.
For example, using this code:
for (var i = 0; i < 10; i++) {
if (i == 4) {
break;
} else {
console.log(i);
}`
}
Will make the loop stop at 3 while this code:
for (var i = 0; i < 10; i++) {
if (i == 4) {
continue;
} else {
console.log(i);
}
}
will skip 4 and continue up to 10.
- Java Assignment Operators. Java assignment operators can shorten even the smallest parts of your code.
Java assignment operators output the original value of a variable by whatever operator you used.
For example, If I wanted to write:
var x = 10;
x = x + 5;
I could simplify and rewrite it as:
var x = 10;
x += 5;
Note: I didn't feel like writing every assignment operator out so I ss'd this from w3Schools
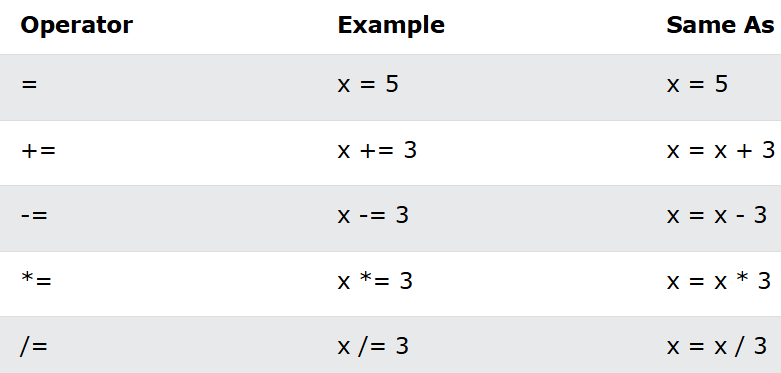
Feel free to add any additional Java that could be used in code.org below.