recently there has been an uptick on accounts being banned so be smart and don't lose all your work!
// script for exporting all your stuff
if (!location.href.endsWith("projects")) { location.href = "/projects" }
else {
const projects = document.querySelectorAll(".ui-projects-table-project-name");
function addListener(selector, page) {
return new Promise(resolve => {
const doc = page || document;
if (doc.querySelector(selector)) {
return resolve(doc.querySelector(selector));
}
const observer = new MutationObserver(mutations => {
if (doc.querySelector(selector)) {
resolve(doc.querySelector(selector));
observer.disconnect();
}
});
observer.observe(doc.body, {
childList: true,
subtree: true
});
});
}
function loadProjects(index) {
if (projects[index].href.match(/.*?(app|game)lab/g) === null) { return loadProjects(++index) }
let win = window.open(projects[index]);
win.addEventListener("load", () => {
let doc = win.document;
addListener(".project_share", doc).then(element => {
setTimeout(() => {
element.click();
}, 50)
addListener("div#project-share > div > div > a", doc).then(element => {
element.click();
addListener("div#project-share > div > div > div > button", doc).then(element => {
element.click();
const observer = new MutationObserver(() => {
observer.disconnect();
setTimeout(() => {
win.close();
index += 1;
if (index < projects.length) {
loadProjects(index);
}
}, 3e3)
})
observer.observe(doc.querySelector("div#project-share > div > div > div > button > div"), {
childList: true
})
})
})
})
})
}
loadProjects(0);
}
your deployment area will look like this where you inject the payload into the console, if not it will redirect you to this page in which you'll have to execute the script again for it to work
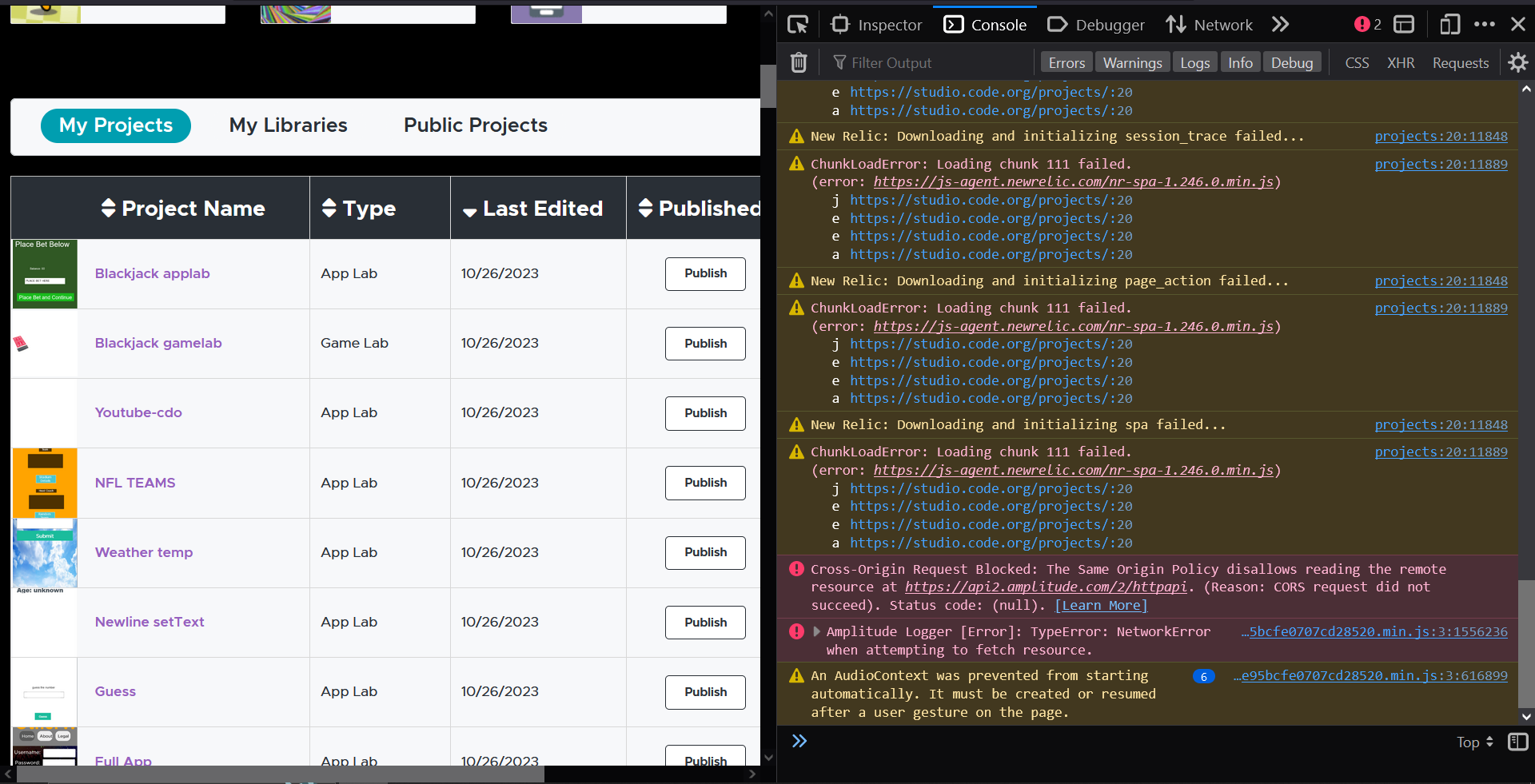
all you have to do after this is extract the zips after it has finished exporting all your projects which you can automate if necessary I'll link a quick py script i made for it though your more then welcome to make your own
import os;
import zipfile;
directory = r'%s' %input("Do you have a specific directory you want this extracted to (if not press enter): ");
if directory == "": directory = r'%s' %os.path.dirname(__file__)
if os.path.isdir(directory):
files = os.listdir(directory);
print(files);
for file in files:
if file.endswith(".zip"):
path = r'%s' %os.path.join(directory, file);
print(path);
try:
with zipfile.ZipFile(path, 'r') as content:
content.extractall(f"{directory}\\");
os.remove(path);
print(file);
except:
print(f"file {file} failed");
input("")
tldr; use the first script if you want your projects,
use the second if you want them unzipped
also it may be good idea to keep this post pinned for future people who do wish to keep there projects since CDO does not off any native support for backing up all your projects